Google Drive API (oauth 2.0) Upload Files Java
Upload Files Java Google Drive
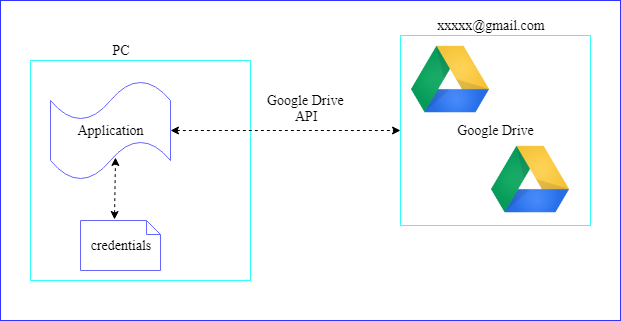
Follow previous :Create Credentials
Add Dependencies to Maven Project
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | <!-- https://mvnrepository.com/artifact/com.google.apis/google-api-services-drive --> <dependency> <groupId>com.google.apis</groupId> <artifactId>google-api-services-drive</artifactId> <version>v3-rev105-1.23.0</version> </dependency> <!-- https://mvnrepository.com/artifact/com.google.api-client/google-api-client --> <dependency> <groupId>com.google.api-client</groupId> <artifactId>google-api-client</artifactId> <version>1.23.0</version> </dependency> <!-- https://mvnrepository.com/artifact/com.google.oauth-client/google-oauth-client-jetty --> <dependency> <groupId>com.google.oauth-client</groupId> <artifactId>google-oauth-client-jetty</artifactId> <version>1.23.0</version> </dependency> |
- Paste client_secret.json file inside the credentials folder
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 | import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.security.GeneralSecurityException; import java.util.Collections; import java.util.List; import com.google.api.client.auth.oauth2.Credential; import com.google.api.client.extensions.java6.auth.oauth2.AuthorizationCodeInstalledApp; import com.google.api.client.extensions.jetty.auth.oauth2.LocalServerReceiver; import com.google.api.client.googleapis.auth.oauth2.GoogleAuthorizationCodeFlow; import com.google.api.client.googleapis.auth.oauth2.GoogleClientSecrets; import com.google.api.client.googleapis.javanet.GoogleNetHttpTransport; import com.google.api.client.http.javanet.NetHttpTransport; import com.google.api.client.json.JsonFactory; import com.google.api.client.json.jackson2.JacksonFactory; import com.google.api.client.util.store.FileDataStoreFactory; import com.google.api.services.drive.Drive; import com.google.api.services.drive.DriveScopes; import com.google.api.services.drive.model.File; import com.google.api.services.drive.model.FileList; public class DriveApp { private static final String APPLICATION_NAME = "Google Drive API Java Quickstart"; private static final JsonFactory JSON_FACTORY = JacksonFactory.getDefaultInstance(); // Directory to store user credentials for this application. private static final java.io.File CREDENTIALS_FOLDER // = new java.io.File(System.getProperty("user.home"), "credentials"); private static final String CLIENT_SECRET_FILE_NAME = "client_secret.json"; // // Global instance of the scopes required by this quickstart. If modifying these // scopes, delete your previously saved credentials/ folder. // private static final List<String> SCOPES = Collections.singletonList(DriveScopes.DRIVE); private static Credential getCredentials(final NetHttpTransport HTTP_TRANSPORT) throws IOException { java.io.File clientSecretFilePath = new java.io.File(CREDENTIALS_FOLDER, CLIENT_SECRET_FILE_NAME); if (!clientSecretFilePath.exists()) { throw new FileNotFoundException("Please copy " + CLIENT_SECRET_FILE_NAME // + " to folder: " + CREDENTIALS_FOLDER.getAbsolutePath()); } // Load client secrets. InputStream in = new FileInputStream(clientSecretFilePath); GoogleClientSecrets clientSecrets = GoogleClientSecrets.load(JSON_FACTORY, new InputStreamReader(in)); // Build flow and trigger user authorization request. GoogleAuthorizationCodeFlow flow = new GoogleAuthorizationCodeFlow.Builder(HTTP_TRANSPORT, JSON_FACTORY, clientSecrets, SCOPES).setDataStoreFactory(new FileDataStoreFactory(CREDENTIALS_FOLDER)) .setAccessType("offline").build(); return new AuthorizationCodeInstalledApp(flow, new LocalServerReceiver()).authorize("user"); } public static void main(String[] args) throws IOException, GeneralSecurityException { System.out.println("CREDENTIALS_FOLDER: " + CREDENTIALS_FOLDER.getAbsolutePath()); // 1: Create CREDENTIALS_FOLDER if (!CREDENTIALS_FOLDER.exists()) { CREDENTIALS_FOLDER.mkdirs(); System.out.println("Created Folder: " + CREDENTIALS_FOLDER.getAbsolutePath()); System.out.println("Copy file " + CLIENT_SECRET_FILE_NAME + " into folder above.. and rerun this class!!"); return; } // 2: Build a new authorized API client service. final NetHttpTransport HTTP_TRANSPORT = GoogleNetHttpTransport.newTrustedTransport(); // 3: Read client_secret.json file & create Credential object. Credential credential = getCredentials(HTTP_TRANSPORT); // 5: Create Google Drive Service. Drive service = new Drive.Builder(HTTP_TRANSPORT, JSON_FACTORY, credential) // .setApplicationName(APPLICATION_NAME).build(); // Print the names and IDs for up to 10 files. FileList result = service.files().list().setPageSize(10).setFields("nextPageToken, files(id, name)").execute(); List<File> files = result.getFiles(); if (files == null || files.isEmpty()) { System.out.println("No files found."); } else { System.out.println("Files:"); for (File file : files) { System.out.printf("%s (%s)\n", file.getName(), file.getId()); } } } } |
Log with gmail account which used to create client_secret.json credentials
Upload.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 | import java.io.IOException; import java.io.InputStream; import java.util.Arrays; import java.util.List; import javax.servlet.RequestDispatcher; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.http.Part; import com.google.api.client.http.AbstractInputStreamContent; import com.google.api.client.http.ByteArrayContent; import com.google.api.client.http.FileContent; import com.google.api.client.http.InputStreamContent; //import com.google.api.client.http.MultipartContent.Part; import com.google.api.services.drive.Drive; import com.google.api.services.drive.model.File; /** * Servlet implementation class Upload */ @WebServlet("/") public class Upload extends HttpServlet { private static final long serialVersionUID = 1L; /** * Default constructor. */ public Upload() { // TODO Auto-generated constructor stub } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse * response) */ private static File _createGoogleFile( String googleFolderIdParent, String contentType, // String customFileName, AbstractInputStreamContent uploadStreamContent) throws IOException { File fileMetadata = new File(); fileMetadata.setName(customFileName); List<String> parents = Arrays.asList(googleFolderIdParent); fileMetadata.setParents(parents); // Drive driveService = GoogleDriveUtils.getDriveService(); File file = driveService.files() .create(fileMetadata, uploadStreamContent) .setFields("id, webContentLink, webViewLink, parents") .execute(); return file; } // Create Google File from byte[] public static File createGoogleFile(String googleFolderIdParent, String contentType, // String customFileName, byte[] uploadData) throws IOException { // AbstractInputStreamContent uploadStreamContent = new ByteArrayContent( contentType, uploadData); // return _createGoogleFile(googleFolderIdParent, contentType, customFileName, uploadStreamContent); } // Create Google File from java.io.File public static File createGoogleFile(String googleFolderIdParent, String contentType, // String customFileName, java.io.File uploadFile) throws IOException { // AbstractInputStreamContent uploadStreamContent = new FileContent( contentType, uploadFile); // return _createGoogleFile(googleFolderIdParent, contentType, customFileName, uploadStreamContent); } // Create Google File from InputStream public static File createGoogleFile(String googleFolderIdParent, String contentType, // String customFileName, InputStream inputStream) throws IOException { // AbstractInputStreamContent uploadStreamContent = new InputStreamContent( contentType, inputStream); // return _createGoogleFile(googleFolderIdParent, contentType, customFileName, uploadStreamContent); } /* * public void path(Part part){ * * } */ private String retrieveFileName(Part part) { String contentDisposition = part.getHeader("content-disposition"); String[] items = contentDisposition.split(";"); for (String str : items) { if (str.trim().startsWith("filename")) { return str.substring(str.indexOf("=") + 2, str.length() - 1); } } return ""; } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse * response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { /* * for (Part part : request.getParts()) { String fileName = * retrieveFileName(part); } */ String path = request.getParameter("fileUpload").replace("\\", "/"); System.out.println("path" + path); // java.io.File uploadFile = new // java.io.File("C:/Users/kasun_000/Desktop/Assign2_SSD/test.txt"); java.io.File uploadFile = new java.io.File(path); // Create Google File: File googleFile = createGoogleFile(null, "text/plain", "newfile.txt", uploadFile); System.out.println("Created Google file!"); System.out.println("WebContentLink: " + googleFile.getWebContentLink()); System.out.println("WebViewLink: " + googleFile.getWebViewLink()); System.out.println("Done!"); RequestDispatcher dispatcher = request .getRequestDispatcher("fileuploadResponse.jsp"); dispatcher.forward(request, response); // response.sendRedirect("/fileuploadResponse.jsp"); } } |
index.jsp
Thank you saivenkat
ReplyDelete